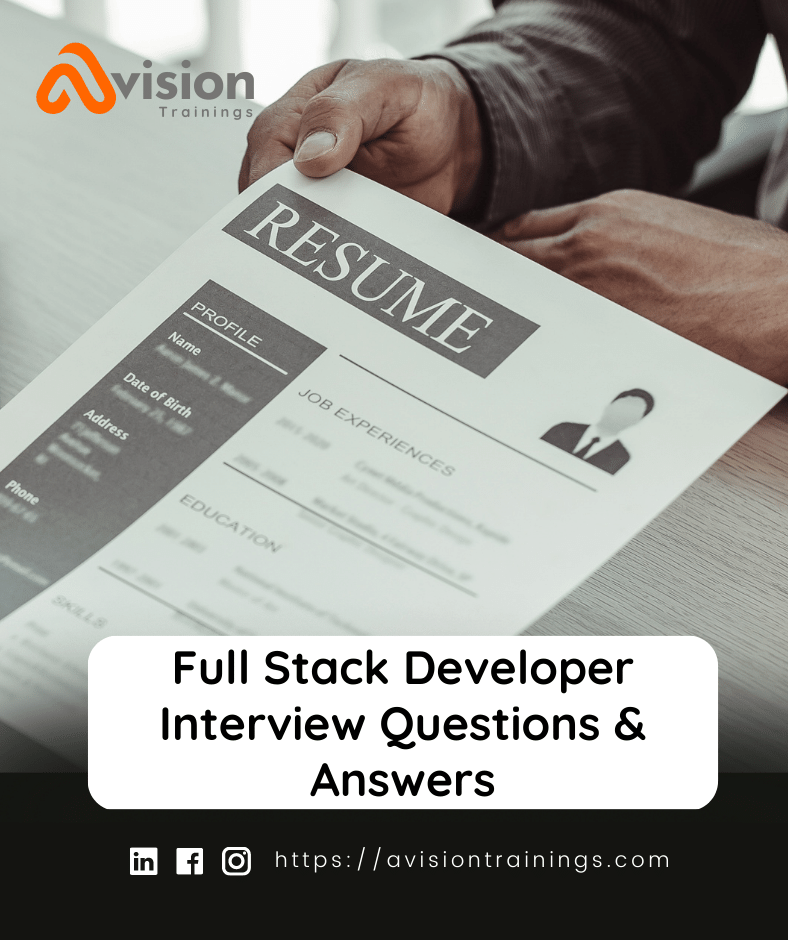
- by Admin
- Rating:
Full Stack Developer Interview Questions and Answers
1. What is the difference between server-side rendering and client-side rendering?
Server-side rendering is the process of generating HTML on the server and sending it to the client. Client-side rendering is the process of generating HTML on the client side using JavaScript. SSR is better for performance and SEO, while CSR provides a better user experience.2. What is version control?
Version control is a system that tracks changes to files and directories over time. It is used to manage and coordinate work on software projects. allowing multiple developers to work on the same codebase without conflicts.3. How do we optimize the performance of a web application?
To optimize the performance of a web application, We have to use techniques such as- Optimizing images
- Minifying CSS JavaScript files
- Caching
- Minimizing HTTP requests
- Compressing files
4. What is the difference between agile and waterfall methodologies?
Waterfall is a traditional software development methodology that follows a sequential process. Each stage is completed before moving on to the next stage. Agile is an iterative and incremental methodology. It focuses on faster delivery of working software with feedback loops and continuous improvement.5. How do we optimize database queries?
Database query optimization involves analyzing and optimizing the SQL statements. It is used to retrieve data from the database. This optimization involves techniques like using indexes, minimizing the number of joins, avoiding subqueries, and using query caching.6. What is a RESTful API?
A RESTful API is an architectural style for building web services. It uses HTTP requests to GET, POST, PUT, and DELETE data. RESTful API is a stateless protocol where each request contains all the necessary information to complete it.7. What is the difference between SQL and NoSQL databases?
SQL databases are relational databases that store data in tables with rows and columns. It uses structured query language (SQL) to manipulate and query data. NoSQL databases are non-relational databases that store data in documents or keyvalue pairs. It uses non-structured query languages to manipulate and query data.8. What is containerization?
Containerization is a technique used to package software in a portable and isolated environment called a container. It allows applications to run consistently across different environments. Containers are lightweight and use fewer resources than traditional virtual machines.9. What is the difference between a primary key and a foreign key in a relational database?
A primary key is a column or set of columns that uniquely identifies each record in a table. Foreign key is a column or set of columns that refers to the primary key of another table. It is used to establish relationships between tables in a relational database.10. How do we handle errors in the code?
Use try/catch blocks to catch and handle errors. In the catch block, log the error and provide an appropriate error message to the user. Use error handling middleware to handle errors that occur during server-side processing.11. What is machine learning and how is it used in web development?
Machine learning is a field of artificial intelligence. It involves building models and algorithms that learn from data and make predictions or decisions. ML is used in web development to improve the user experience, personalize content, and automate tasks. It can be integrated into web applications using APIs or libraries such as TensorFlow and Scikit-learn.12. How do we implement authentication in your web applications?
We can use industry-standard authentication protocols such as OAuth2 and OpenID Connect to authenticate users. We can also use secure session management and implement measures such as password hashing and salting to protect user credentials.13. What is the difference between a front-end and back-end developer?
Front-end developers are responsible for providing an effective user interface and user-friendly experience of a web application. They use HTML, CSS, and JavaScript to create interactive web pages. Back-end developers are responsible for the serverside of a web application. They use programming languages such as PHP, Ruby, Python, or Java to create the logic and functionality of the application.14. What is micro services architecture?
Micro services architecture is an approach to software development. It allows applications to be built as a collection of small, independently deployable services. Each service performs a specific function and communicates with other services using lightweight protocols such as HTTP or message queues. Micro services architecture allows for greater flexibility, scalability, and resiliency than monolithic architectures.15. How do we ensure the security of a web application?
To ensure the security of a web application, we can use techniques such as input validation, sanitization, and encryption. Also we can use HTTPS to secure the communication between the client and server. Implement measures such as access control, firewalls, and intrusion detection systems.16. What is the difference between a GET request and a POST request?
A GET request is used to retrieve data from a server, while a POST request is used to send data to a server to be processed. GET requests can be cached by the browser, while POST requests cannot.17. How do we handle cross-site scripting (XSS) attacks in web applications?
We use input validation and sanitization to prevent XSS attacks. We also use Content Security Policy (CSP) to restrict the sources of content. It can be loaded by a page, preventing malicious scripts from running.18. What is the difference between web sockets and HTTP requests?
HTTP requests are used to send and receive data between the client and server in a request-response format. Web sockets provide a persistent connection between the client and server, allowing real-time communication between the two.19. What is DevOps?
DevOps is a software development methodology that combines development (Dev) and operations (Ops) to create a culture of collaboration and automation. It involves the use of tools and processes to automate the building, testing, and deployment of software. DevOps ensures the goal of delivering high-quality software faster and more reliably.20. What is the difference between synchronous and asynchronous programming?
Synchronous programming is when code executes one line at a time and waits for each line to finish before moving on to the next. Asynchronous programming is when code executes without blocking, allowing other code to run while it's waiting for an operation to finish. Asynchronous programming is more efficient for tasks such as network requests and I/O operations.21. If the task is to create a web application that allows users to create and edit documents, What technologies and tools would you use to accomplish this task?
To complete this task, We have to use the following technologies and tools:- Front-end: React.js for building the user interface
- Back-end: Node.js and Express.js for building the server-side application
- Database: MongoDB for storing the documents
- Other tools: Git for version control, and Postman for testing the API endpoints
22. The new project is to add a new feature for an existing web application. What steps are needed to ensure a smooth integration and minimize the impact on the existing application?
To ensure a smooth integration and minimize the impact on the existing application, the following steps are needed:- Research the API documentation to understand how it works and what data it provides.
- Write tests to verify that the API is working correctly and the data is being retrieved and displayed correctly.
- Use version control to create a new branch for the feature.
- Make sure to document any changes made to the codebase and the API integration process for reference.
- Test the integration in a staging environment before deploying to production to catch any issues before they impact end-users.
23. What is the difference between a framework and a library?
Framework is a pre-built set of tools and guidelines for building applications. It provides a structure and a set of rules for development. Library is a collection of reusable code that can be used to perform specific tasks. They are used to perform specific functions within the structure provided by the framework.24. How to handle authentication and authorization in a web application?
Authentication is the process of verifying the identity of a user. To achieve user authentication, use a secure p assword hashing algorithm. To add security, implement two-factor authentication.Authorization is the process of determining what actions a user is allowed to perform. To achieve user authorization, use access control lists